Building Apps
CalHacks 9.0 Workshop
Mobile Developers of Berkeley
Introduction
Mobile applications continue to be the primary way people interface with technology. When building applications at hackathons, there’s a lot to consider. One of the most important factors is time. You want to build something that’s useful, but you also want to build something that’s feasible. To do so, we will be using React Native to build cross-platform applications and Firebase on the backend, as they allow us to build applications quickly and efficiently.
In this workshop, we will explore React Native and Firebase to build a simple mobile application with authentication and a database. We will start with an overview of React Native fundamentals, followed by a short introduction to Firebase. We will demonstrate how you can quickly prototype and view your app using Expo and Expo Go. You will have the opportunity to follow along using the provided starter code and instructions.
This is a beginner/intermediate level workshop intended to teach the concepts of React and the basics of React Native to build mobile applications, presented by the Mobile Developers of Berkeley VP of Education, Aniruth Narayanan.
Prerequisites
Note: these are not hard requirements. You can always follow along, but some parts may be trickier.
- Programming basics in any language (Python, Java, Javascript, Typescript, C, etc.)
- Conditionals, loops, variables, etc.
- Students currently enrolled in Berkeley’s 61A or equivalent level course should be fine
- Check out the guides on transitioning from Python to Javascript and Java to Javascript!
- Experience using apps of any kind
- Instagram, Snapchat, Messages/iMessage, Camera, etc.
- Setup of dev environment
- See below for things that need to be done before the workshop
- Nice to have but not required:
- Javascript/Typescript familiarity - there are some guides below to help you get started
- Basic web development knowledge - HTML, CSS, JS, though not necessary
- Data Structures or other CS classes (i.e. Berkeley’s CS 61B)
Guides for Transitioning to JavaScript
JavaScript is the language used in React Native. JavaScript Express is a general guide about JavaScript.
If you are familiar with Python or Java, you can use the following guides to help you transition to JavaScript.
These are listed (roughly) in order of depth.
From Python:
- JavaScript for Python Programmers by Thomas Ballinger
- JavaScript for Python Programmers by Mike Deplatis
- JS4Python
From Java:
- Transitioning from Java to JavaScript by Greg Bryne
- JavaScript for Java Developers, Angular University
Most projects use TypeScript, a superset of JavaScript that enforces typing (similar to Java and newer versions of Python, with differences between objects, numbers, strings, etc.). The demo app will be using TypeScript, but all typing information will be specified. It might seem more complicated at first to use TypeScript, but it will help you write more robust code.
Setup
You can use Windows, Mac, or Linux for this workshop.
Note that this setup should be done before the workshop. If you don’t have it, there will be live coding on the screen, and you’re free to do the setup steps and then code later.
Installation
Install VS Code as your code editor.
If on Windows: install git bash.
If on Mac: install xcode-command-line-tools using the command xcode-select --install
.
Install Node, verify node –version
and npm –version
work (they should be printing out versions). Install yarn and confirm it works as well using yarn --version
. Install expo-cli globally using npm i -g expo-cli
(using sudo if on mac if need be).
Install the Expo Go app on your phone. This is how you can test code running on your local machine on the phone, by scanning the QR code that appears in the terminal when running expo start
.
Use git to take the template we have of the starter code (linked below) and clone it locally. Enter the directory (using cd [folder name]
), then run yarn install
and expo install
within the directory.
Verify your setup is correct by running expo start
in the cloned repository. A QR code should pop up in the terminal, and when scanned in Expo Go, should load up the starter app code.
Optional: if on Mac, install XCode to get the simulator tools (so you can open up a simulated iPhone on the screen, instead of having to use your phone) - so the command expo start –-ios
can be used. Note that you can use an Android emulator if on Windows or Linux if you’d like.
To summarize: you should have Node, Yarn, Expo-CLI, Expo Go, and VS Code installed. You should have cloned the starter code and run yarn install
and expo install
within the directory. You should be able to run expo start
and scan the QR code in Expo Go to see the starter app on a phone or simulator.
Firebase Setup
This project will require you to set up a Firebase project and database instance. To do so, follow the instructions below.
- Sign into Firebase using your Google account (you may need to do so from a personal one if your .berkeley.edu email does not work).
- In the Firebase Console, click Add Project, then enter a Project Name (MDB CalHacks Demo).
- Continue through the setup and click Create Project.
- On the project dashboard, select the “Web” option to create a new Web app (you can also do this through the settings page, accessible through the sidebar).
- Enter an App Nickname (call it “Voting App”) and click Register.
- Copy just the
firebaseConfig
variable. Convert it to JSON (which will require adding quotes around the object’s attributes). Store it in a new file calledkeys.json
in your project’s root directory. It should be identical in structure to the file calledkeys.json.template
.
To set up Cloud Firestore (our NoSQL cloud database):
- Click on the Cloud Firestore tab in the sidebar.
- Click Create Database and choose Test Mode when prompted.
- Select any location for your instance and continue to click Done.
To set up Authentication (our user management system):
- Click on the Authentication tab in the sidebar.
- Click Get Started and select Email/Password as your sign-in method.
- Click Enable.
React Native and Firebase
The slides used in the presentation will are available here and below.
For a more in depth intro to building apps (including backend, security, etc.) check out our lessons.
Demo
Every Sunday, the Mobile Developers of Berkeley hosts a weekly meeting where we discuss the latest in mobile development, share our projects, and learn from each other. We provide free lunch for our members, funded by our contracts, except there’s one issue: it’s too hard to decide where to buy food. To solve this, we are going to build a mobile application that allows members to vote on where to get lunch from, using React Native and Firebase.
Voting and Results Screens:
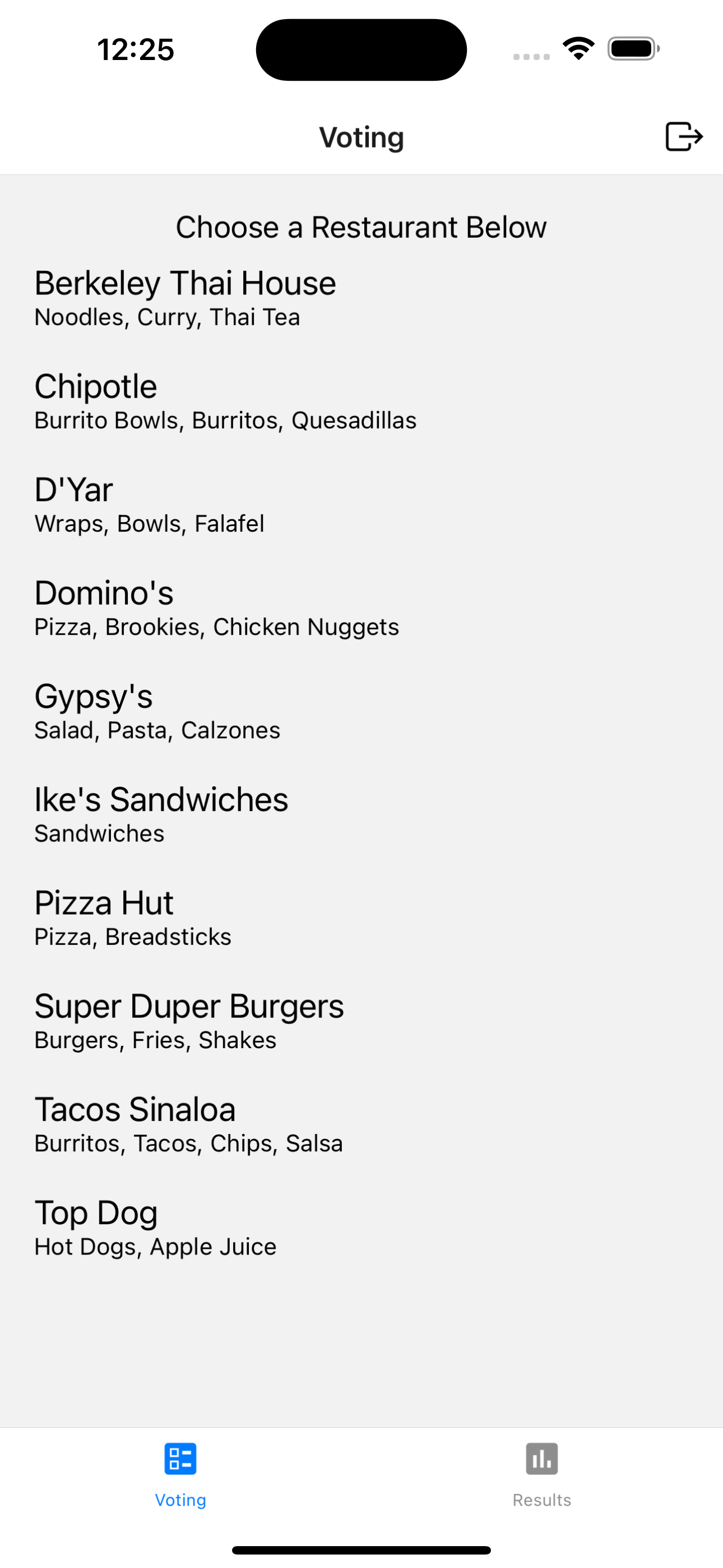
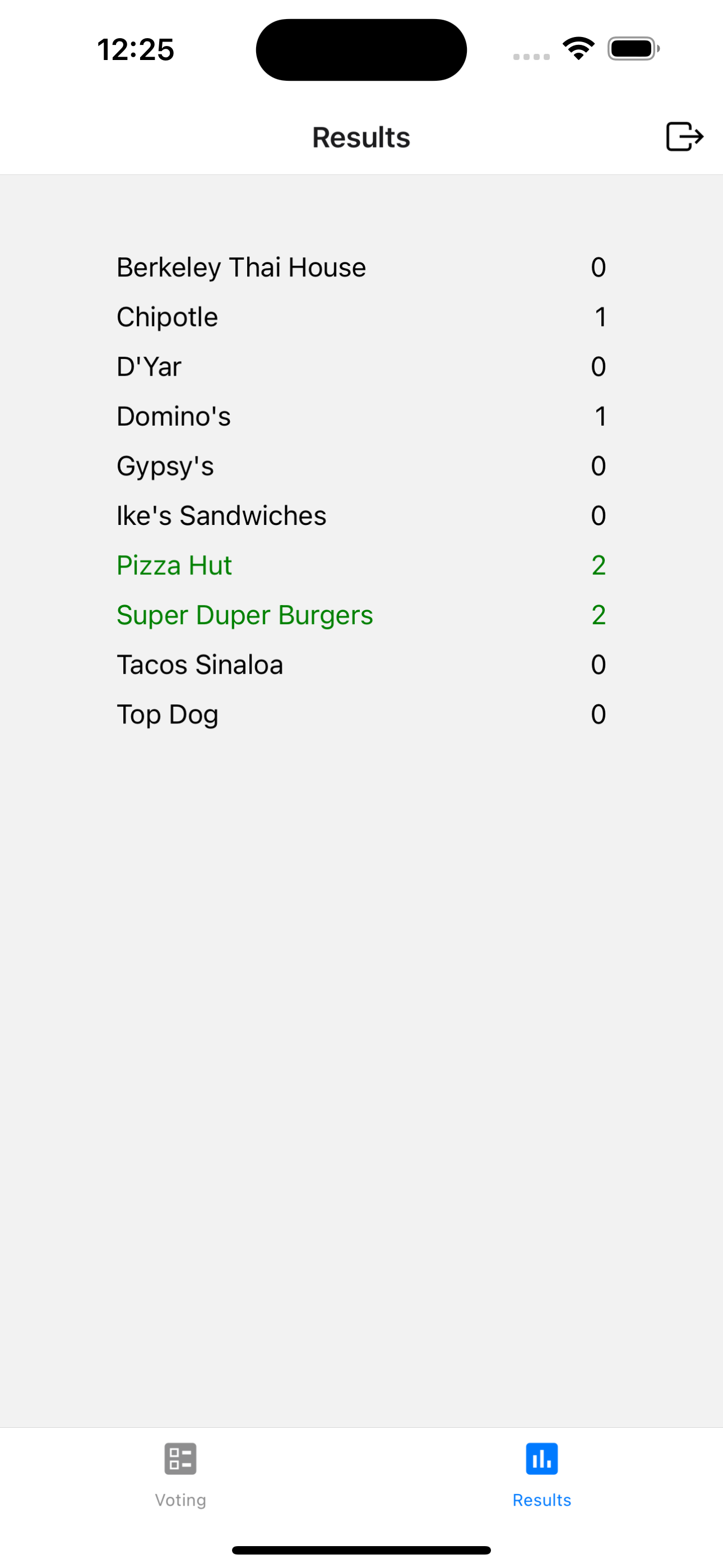
Authentication Screens (Sign In, Sign Up):
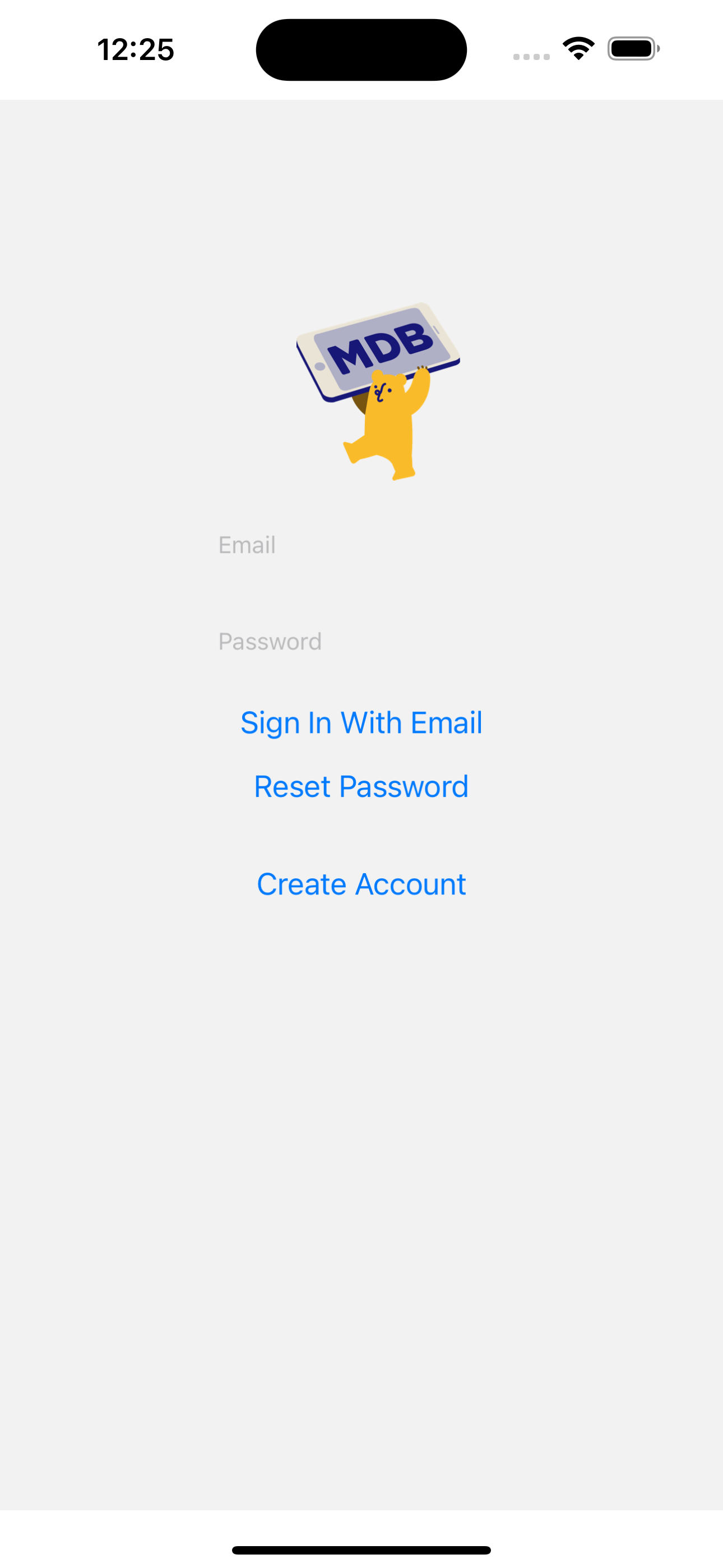
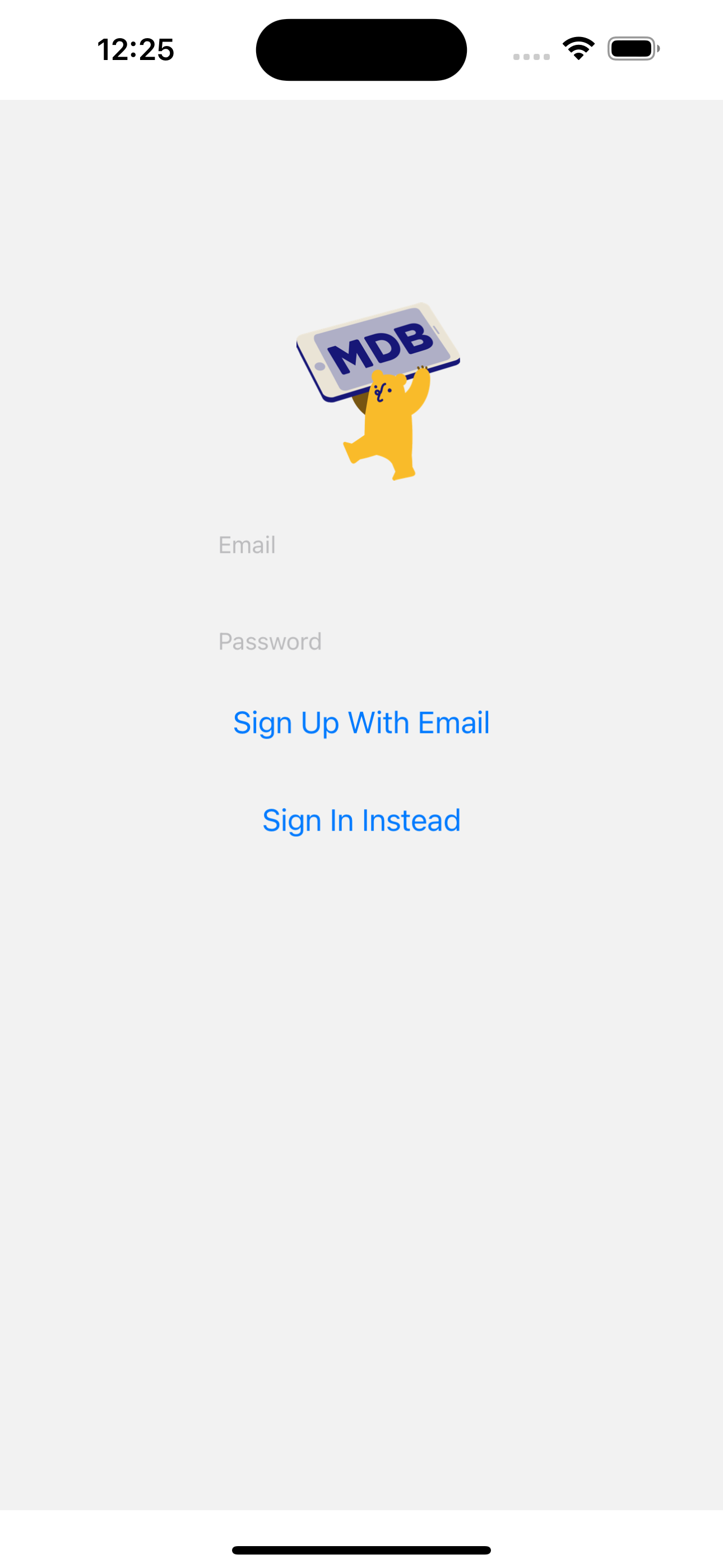
Starter Code
To create your repository, go to the following link:
https://github.com/aniruthn/calhacks9-starter.git
You should see the following “Use this template” button:

After clicking on it, you should see the following:
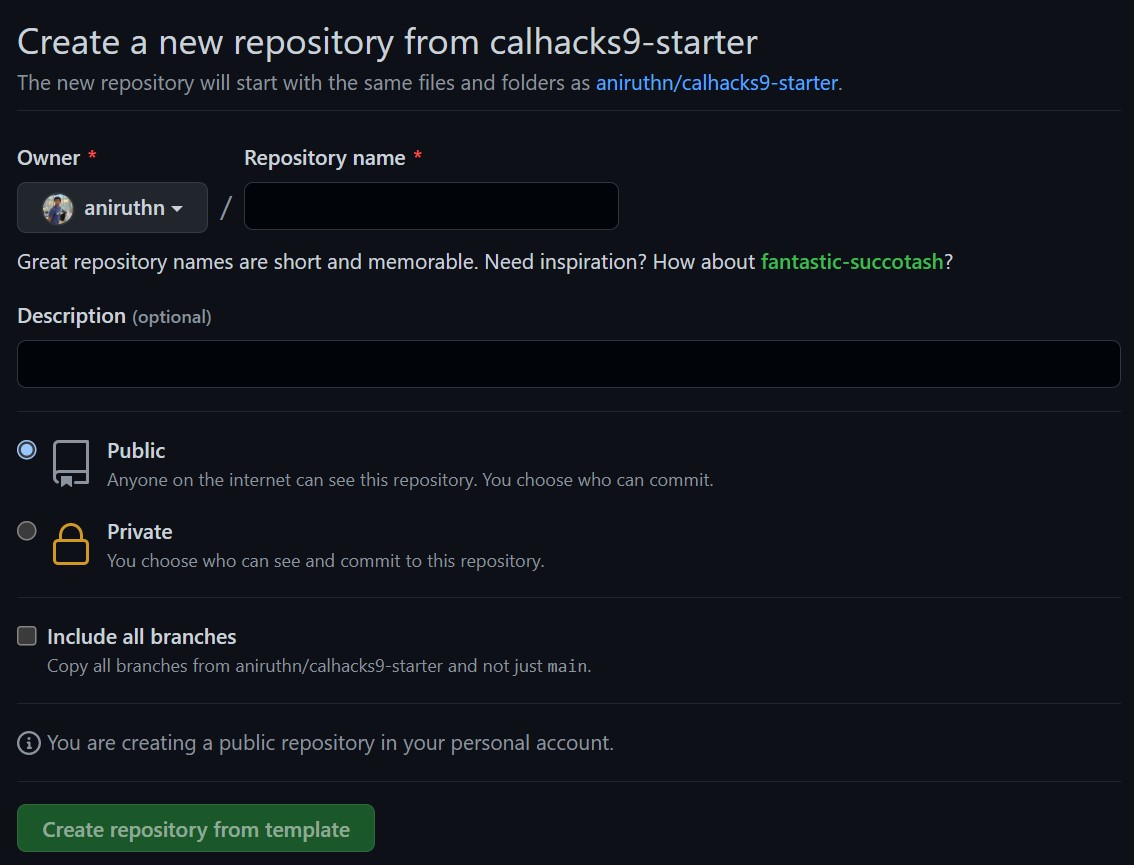
Click on the green “Use this template” button and follow the instructions to create your own repository. Name it whatever you’d like.
Once the templated repository is created, you should see the following:
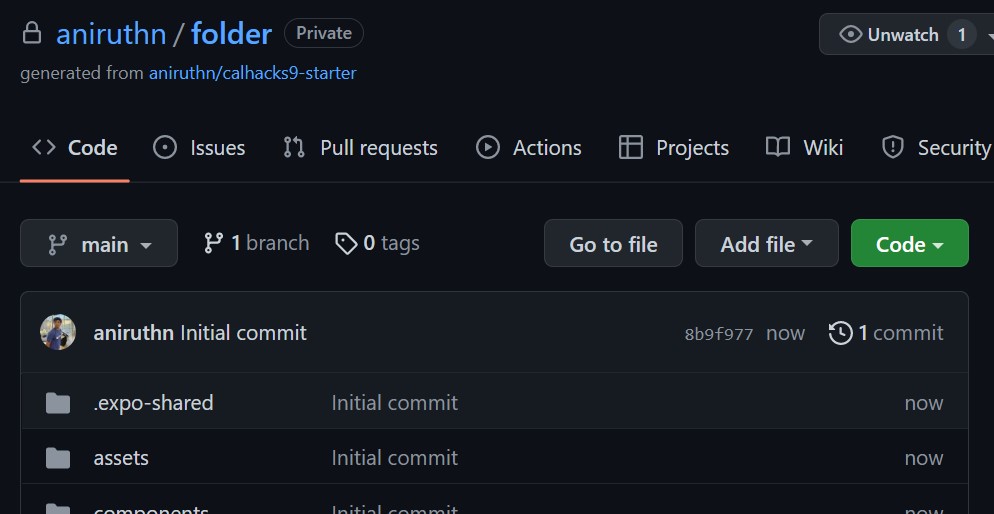
Then, clone that repository (not the template) to your local machine. You can do this by running the following command in your terminal:
git clone [your repository link]
To get the repository link, click on the green “Code” button and copy the link:
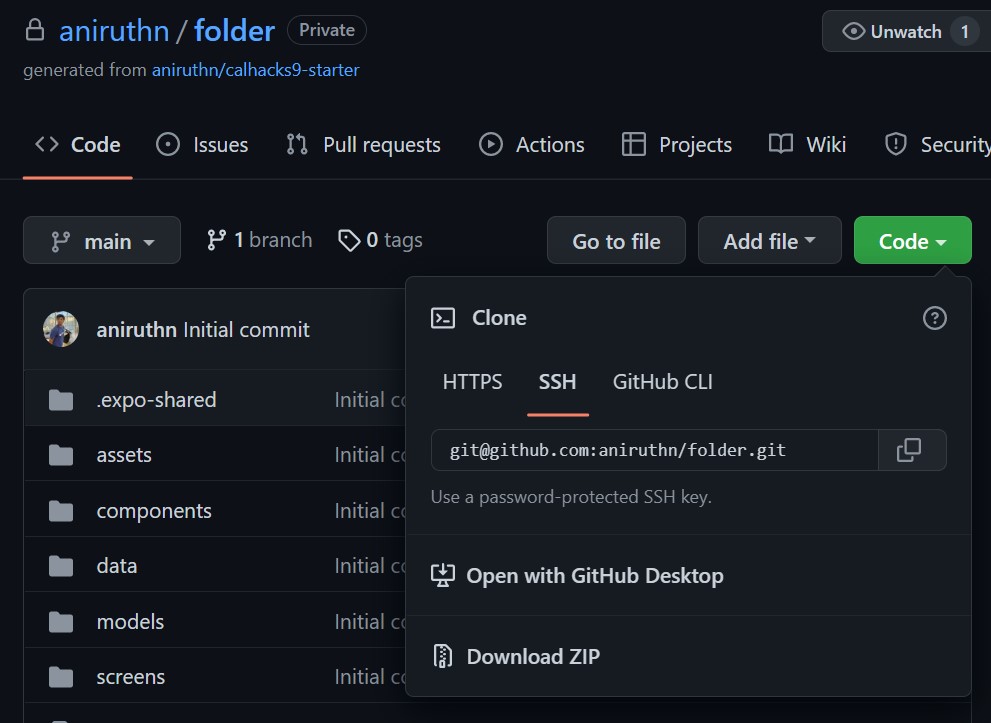
Reference Documentation
While you’re working, it’s helpful to keep an copy of the reference documentation open so you can see examples of components being used.
React Native Component Documentation (can use either):
- https://docs.expo.dev/versions/v46.0.0/react-native
- https://reactnative.dev/docs/components-and-apis
Firebase (use web documentation, since we’re using Javascript in React Native):
- Authentication
- Firestore
- Cloud Storage (not used in this workshop, but useful to store images)